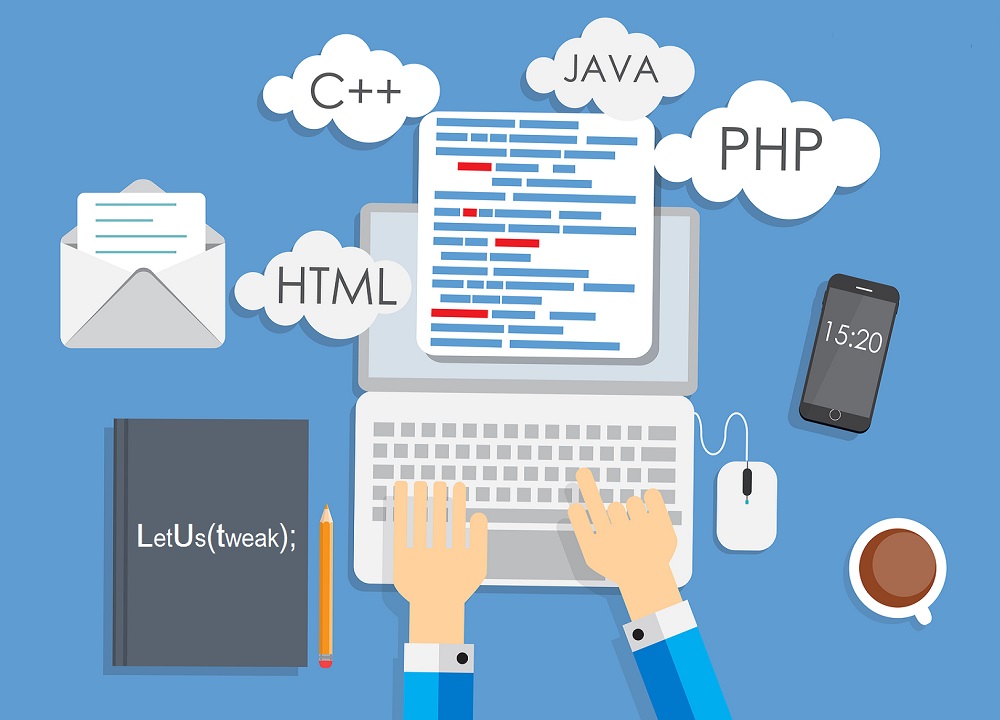
Animated Solar System
Guys, in this article we are going to provide you a cool snippet for developing- Animated Solar System, using only HTML and CSS. Yes only HTML and CSS. So without wasting time let’s go through it..
HTML5 | Snippet
Here’s a HTML code snippet, just put in any editor (i.e. Notepad, Notedpad++, VS etc..) and give it a name as per your choice. But, don’t forget to give it an extension .html / .htm .. In my case it’s Solar_System.html .. Take a look at the code and try it out.
<html>
<head>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<!-- Animated Solar System -->
<!-- Right below is an image of the sun -->
<img id="sun" src="C:\....\sun.jpg">
<!-- Inserting the Planet Images -->
<!-- Insert the 'Mercury' on the next line -->
<div id='mercury-orbit'>
<img id="mercury" src="C:\....\mercury.jpg"> </div>
<!-- Insert the 'Venus' image source on the next line -->
<div id='venus-orbit'>
<img id="venus" src="C:\....\venus.jpg"> </div>
<!-- Insert the 'Earth' image source on the next line -->
<div id='earth-orbit'>
<img id="earth" src="C:\....\earth.jpg"> </div>
<!-- Insert the 'Mars' image source on the next line -->
<div id='mars-orbit'>
<img id="mars" src="C:\....\mars.jpg"> </div>
<!-- Insert the 'Jupiter' image source on the next line -->
<div id='jupiter-orbit'>
<img id="jupiter" src="C:\....\jupiter.jpg"> </div>
<!-- Insert the 'Saturn' image source on the next line -->
<div id='saturn-orbit'>
<img id="saturn" src="C:\....\saturn.jpg"> </div>
<!-- Insert the 'Uranus' image source on the next line -->
<div id='uranus-orbit'>
<img id="uranus" src="C:\....\uranus.jpg"> </div>
<!-- Insert the 'Neptune' image source on the next line -->
<div id='neptune-orbit'>
<img id="neptune" src="C:\....\neptune.jpg"> </div>
</body>
</html>
Points to Remember- Add the image locations as per your machine and try to select the images with Black backgrounds.
CSS3 | Snippet
This part is the main part of this article. In this, I’ll show you how to animate things using CSS. So guys, go through this part but be attentive while using and try this. Because, a single minute mistake can ruin everything. Have a look..
/* Animated Solar System */
html, body {
/* The Universe takes up all available space - Black */
/* That's why I suggested choice the images with black background */
width: 100%;
height: 100%;
/* The universe is black */
/* That's why I suggested choice the images with black background */
background-color: black;
/* Properties for the Sun */
#sun {
position: absolute;
top: 50%;
left: 50%;
height: 200px;
width: 200px;
margin-top: -100px;
margin-left: -100px;
}
/* End of Sun's Properties */
/* Properties for the Mercury */
#mercury {
position: absolute;
top: 0%;
left: 45%;
height: 20px;
width: 20px;
margin-left: -10px;
margin-top: -10px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#mercury-orbit {
position: absolute;
top: 60%;
left: 57%;
width: 300px;
height: 300px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Mercury - Rotation and Speed */
-webkit-animation: spin-right 5s linear infinite;
-moz-animation: spin-right 5s linear infinite;
-ms-animation: spin-right 5s linear infinite;
-o-animation: spin-right 5s linear infinite;
animation: spin-right 5s linear infinite;
}
/* End of Mercury's Properties */
/* Properties for the Venus */
#venus {
position: absolute;
top: 0%;
left: 45%;
height: 30px;
width: 30px;
margin-left: -15px;
margin-top: -15px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#venus-orbit {
position: absolute;
top: 55%;
left: 53%;
width: 400px;
height: 400px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Venus - Rotation and Speed */
-webkit-animation: spin-right 5s linear infinite;
-moz-animation: spin-right 5s linear infinite;
-ms-animation: spin-right 5s linear infinite;
-o-animation: spin-right 5s linear infinite;
animation: spin-right 5s linear infinite;
}
/* End of Venus's Properties */
/* Properties for the Earth */
#earth {
position: absolute;
top: 0;
left: 50%;
height: 30px;
width: 30px;
margin-left: -20px;
margin-top: -20px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#earth-orbit {
position: absolute;
top: 45%;
left: 50%;
width: 500px;
height: 500px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Earth - Rotation and Speed */
-webkit-animation: spin-right 10s linear infinite;
-moz-animation: spin-right 10s linear infinite;
-ms-animation: spin-right 10s linear infinite;
-o-animation: spin-right 10s linear infinite;
animation: spin-right 10s linear infinite;
}
/* End of Earth's Properties */
/* Properties for the Mars */
#mars {
position: absolute;
top: 0%;
left: 45%;
height: 20px;
width: 20px;
margin-left: -10px;
margin-top: -10px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#mars-orbit {
position: absolute;
top: 40%;
left: 46%;
width: 600px;
height: 600px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Mars - Rotation and Speed */
-webkit-animation: spin-right 15s linear infinite;
-moz-animation: spin-right 15s linear infinite;
-ms-animation: spin-right 15s linear infinite;
-o-animation: spin-right 15s linear infinite;
animation: spin-right 15s linear infinite;
}
/* End of Mars' Properties */
/* Properties for the Jupiter */
#jupiter {
position: absolute;
top: 0%;
left: 45%;
height: 70px;
width: 70px;
margin-left: -28px;
margin-top: -28px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#jupiter-orbit {
position: absolute;
top: 35%;
left: 43%;
width: 700px;
height: 700px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Jupiter - Rotation and Speed */
-webkit-animation: spin-right 20s linear infinite;
-moz-animation: spin-right 20s linear infinite;
-ms-animation: spin-right 20s linear infinite;
-o-animation: spin-right 20s linear infinite;
animation: spin-right 20s linear infinite;
}
/* End of Jupiter's Properties */
/* Properties for the Saturn */
#saturn {
position: absolute;
top: 0%;
left: 45%;
height: 70px;
width: 70px;
margin-left: -28px;
margin-top: -28px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#saturn-orbit {
position: absolute;
top: 30%;
left: 38%;
width: 800px;
height: 800px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Saturn - Rotation and Speed */
-webkit-animation: spin-right 25s linear infinite;
-moz-animation: spin-right 25s linear infinite;
-ms-animation: spin-right 25s linear infinite;
-o-animation: spin-right 25s linear infinite;
animation: spin-right 25s linear infinite;
}
/* End of Saturn's Properties */
/* Properties for the Uranus */
#uranus {
position: absolute;
top: 0%;
left: 45%;
height: 50px;
width: 50px;
margin-left: -25px;
margin-top: -25px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#uranus-orbit {
position: absolute;
top: 18%;
left: 32%;
width: 1000px;
height: 1000px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Uranus - Rotation and Speed */
-webkit-animation: spin-right 30s linear infinite;
-moz-animation: spin-right 30s linear infinite;
-ms-animation: spin-right 30s linear infinite;
-o-animation: spin-right 30s linear infinite;
animation: spin-right 30s linear infinite;
}
/* End of Uranus' Properties */
/* Properties for the Neptune */
#neptune{
position: absolute;
top: 0%;
left: 45%;
height: 50px;
width: 50px;
margin-left: -25px;
margin-top: -25px;
}
/* Properties for - Position / Orbit / Radius / Rotation */
#neptune-orbit {
position: absolute;
top: 8%;
left: 26%;
width: 1200px;
height: 1200px;
margin-top: -250px;
margin-left: -250px;
border-width: 2px;
border-style: dotted;
border-color: white;
border-radius: 50%;
/* Animating Neptune - Rotation and Speed */
-webkit-animation: spin-right 35s linear infinite;
-moz-animation: spin-right 35s linear infinite;
-ms-animation: spin-right 35s linear infinite;
-o-animation: spin-right 35s linear infinite;
animation: spin-right 35s linear infinite;
}
/* End of Neptune's Properties */
/* Keyframes for Roatation - Right */
@-webkit-keyframes spin-right {
100% {
-webkit-transform: rotate(360deg);
-moz-transform: rotate(360deg);
-ms-transform: rotate(360deg);
-o-transform: rotate(360deg);
transform: rotate(360deg);
}
}
@keyframes spin-right {
100% {
-webkit-transform: rotate(360deg);
-moz-transform: rotate(360deg);
-ms-transform: rotate(360deg);
-o-transform: rotate(360deg);
transform: rotate(360deg);
}
}
Points to Remember-
Don’t make unnecessary changes in the code such as- properties for animations, rotation. In addition, to that keep the statements as they are like- if they are in small letters then keep them in that or else you need to make some other changes in the CSS file as well as HTML file. One more thing, save the file with the name- style.css (In case you are changing the name of the CSS file, then don’t forget to change that name in HTML file as well.)
I hope it help guys. For more info or any doubt right back to us. Keep Visitng, for more tech stuff. Keep Sharing & Tweaking! 🙂